- Frugal Cafe
- Posts
- FC39: The best ways to generate strings
FC39: The best ways to generate strings
Not allocate a new string, or single allocation and single pass copying

The best way to generate strings is not generating a new string when reasonably possible.
The second-best way to generate strings is calculating exact length, allocating once, then copying source data in a single pass.
Here are a few implementations which achieve such gold standard:


Notice in Concat(string, string), if one string is null or empty, the other string is returned without creating a new string. Concat(string, string, string) can achieve the same. If new string allocation is needed, there is just a single allocation, and single pass copying from source to new string.
This is totally different if you’re using StringBuilder or ValueStringBuilder to generate string. Strings are always allocated, except when zero length, and data is copied into char buffer first.
Here is a method meeting the second rule, but missing the first one:

string.Join should return the first string when count is 1. This issues seems to have been fixed in .Net Core.
Notice all these methods are calling internal method string.FastAllocateString to allocate new string, then use unsafe code to fill string content. For user allocation, you can use new string(char.MinValue, length) to get similar allocation efficiency.
Sample usage:

.Net Core introduces a new string allocation API for such pattern:
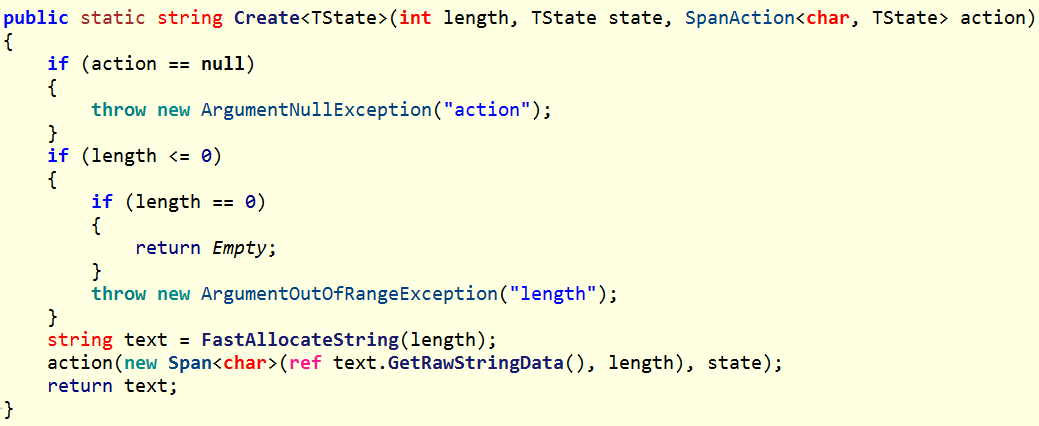
You can use Action delegate on Span to avoid using unsafe code. But please make sure the delegate usage is not causing extra allocations.
Sample usage:
